ICPC冬令营Day02
指针+简单计算几何
E - Satellites
The radius of earth is 6440 Kilometer. There are many Satellites and Asteroids moving around the earth. If two Satellites create an angle with the center of earth, can you find out the distance between them? By distance we mean both the arc and chord distances. Both satellites are on the same orbit (However, please consider that they are revolving on a circular path rather than an elliptical path).
Input
The input file will contain one or more test cases. Each test case consists of one line containing two-integer s and a, and a string ‘min’ or ‘deg’. Here s is the distance of the satellite from the surface of the earth and a is the angle that the satellites make with the center of earth. It may be in minutes (′ ) or in degrees (◦ ). Remember that the same line will never contain minute and degree at a time.
Output
For each test case, print one line containing the required distances i.e. both arc distance and chord distance respectively between two satellites in Kilometer. The distance will be a floating-point value with six digits after decimal point.
Sample Input
500 30 deg
700 60 min
200 45 deg
Sample Output
3633.775503 3592.408346
124.616509 124.614927
5215.043805 5082.035982
题目分析:
给出圆心角以及半径,求出两个卫星之间的弦长以及弧长
根据弧长公式:弧长 = 2Πr\(角度)/360
根据弦长公式:弦长 = 2r\sin(角度*Π/(2*180))
所以只要处理好题目中说的角度以及分就可以了,如果是分的话就在原来的基础上除60(1°=60′)
如果夹角超过180那就用360减掉原来的度数即可
AC代码:
#include<iostream>
#include<cmath>
using namespace std;
double s,a;
string op;
//acos(-1)表示Π
int main(){
while(cin >> s >> a >> op){
if(op == "min") a /= 60;
if(a > 180) a = 360 - a;
double arcdist = 2*acos(-1)*(s+6440.0)*a/360;
double chord_dist = (s + 6440.0)*sin(a*acos(-1)/360)*2;
printf("%.6lf %.6lf\n",arcdist,chord_dist);
}
return 0;
}
G - The Circumference of the Circle
To calculate the circumference of a circle seems to be an easy task - provided you know its diameter. But what if you don’t?
You are given the cartesian coordinates of three non-collinear points in the plane.
Your job is to calculate the circumference of the unique circle that intersects all three points.
Input
The input will contain one or more test cases. Each test case consists of one line containing six real numbers x1,y1, x2,y2,x3,y3, representing the coordinates of the three points. The diameter of the circle determined by the three points will never exceed a million. Input is terminated by end of file.
Output
For each test case, print one line containing one real number telling the circumference of the circle determined by the three points. The circumference is to be printed accurately rounded to two decimals. The value of pi is approximately 3.141592653589793.
Sample Input
0.0 -0.5 0.5 0.0 0.0 0.5
0.0 0.0 0.0 1.0 1.0 1.0
5.0 5.0 5.0 7.0 4.0 6.0
0.0 0.0 -1.0 7.0 7.0 7.0
50.0 50.0 50.0 70.0 40.0 60.0
0.0 0.0 10.0 0.0 20.0 1.0
0.0 -500000.0 500000.0 0.0 0.0 500000.0
Sample Output
3.14
4.44
6.28
31.42
62.83
632.24
3141592.65
题目分析:
三角形的三个点的坐标,三点共圆,求出这个圆的周长,根据海伦公式+正弦定理,可以求出来该圆的直径
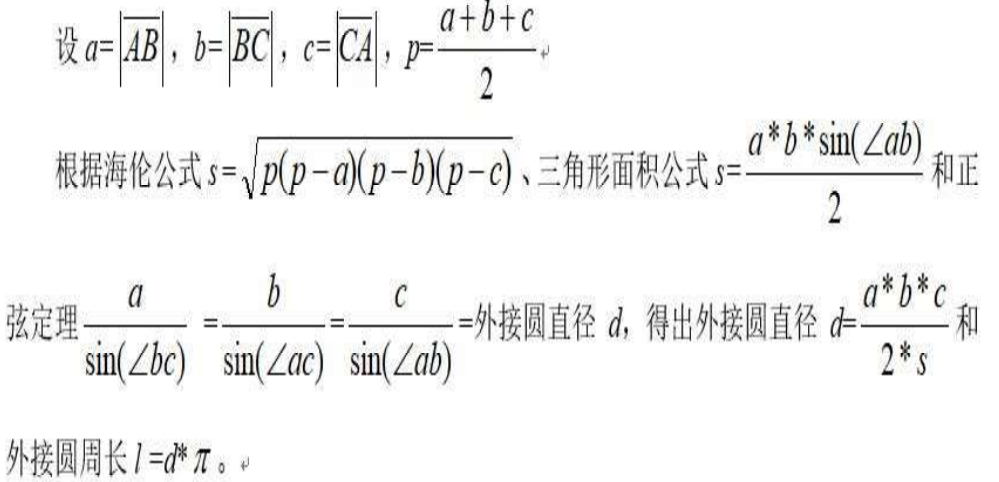
AC代码:
#include<iostream>
#include<cmath>
#include<cstdio>
using namespace std;
double xa,ya,xb,yb,xc,yc,p;
double getDistance(double x,double y,double xx,double yy){
return sqrt(pow(x-xx,2)+pow(y-yy,2));
}
int main(){
while(cin >> xa >> ya >> xb >> yb >> xc >> yc){
double a = getDistance(xa,ya,xb,yb);
double b = getDistance(xb,yb,xc,yc);
double c = getDistance(xa,ya,xc,yc);
double p = (a+b+c)/2;
double s = sqrt(p*(p-a)*(p-b)*(p-c));
double d = a*b*c/2.0/s;
printf("%.2f\n",d*acos(-1.0));
}
return 0;
}
- 本文链接:http://yoursite.com/2021/01/19/ICPC%E5%86%AC%E4%BB%A4%E8%90%A5Day02/
- 版权声明:本博客所有文章除特别声明外,均默认采用 许可协议。
若没有本文 Issue,您可以使用 Comment 模版新建。
GitHub IssuesGitHub Discussions